Tolgee for Next
Translate Next.js apps with Tolgee the way you want. Tolgee offers powerful native SDKs with in-context editing, screenshot generation, or context extraction for AI translator. If you prefer to manage the strings another way, Tolgee provides a powerful REST API.
Placeholders & HTML
No more confused translators with specific placeholder and markup syntax. Placeholders and HTML tags are visualized in the Tolgee Platform.
Placeholders & HTML
No more confused translators with specific placeholder and markup syntax. Placeholders and HTML tags are visualized in the Tolgee Platform.
Open-source & self-hosted
Tolgee is open-source and can be self-hosted. Keep your data safe if your policies require it.
Open-source & self-hosted
Tolgee is open-source and can be self-hosted. Keep your data safe if your policies require it.
Cross-platform
Reuse the same strings on mobile, web or backend. What's stored in Tolgee can be exported to any supported format.
Cross-platform
Reuse the same strings on mobile, web or backend. What's stored in Tolgee can be exported to any supported format.
AI Translator
Get reliable results from the Tolgee AI translator. It uses all the data possible to provide the best results.
AI Translator
Get reliable results from the Tolgee AI translator. It uses all the data possible to provide the best results.
Simple & Delightful UI
Tolgee's UI is so simple that you won't get lost in it. We prioritize delightful and clean UI when we design any feature.
Simple & Delightful UI
Tolgee's UI is so simple that you won't get lost in it. We prioritize delightful and clean UI when we design any feature.
Placeholders & HTML
No more confused translators with specific placeholder and markup syntax. Placeholders and HTML tags are visualized in the Tolgee Platform.
Do it your way
Tolgee native SDK
Tolgee native SDK provide the best developer and translator experience. Enhance in-context editing, context extraction or one-click screenshot generation.
TOLGEE CLI
Import & Export via UI
REST API & Webhooks
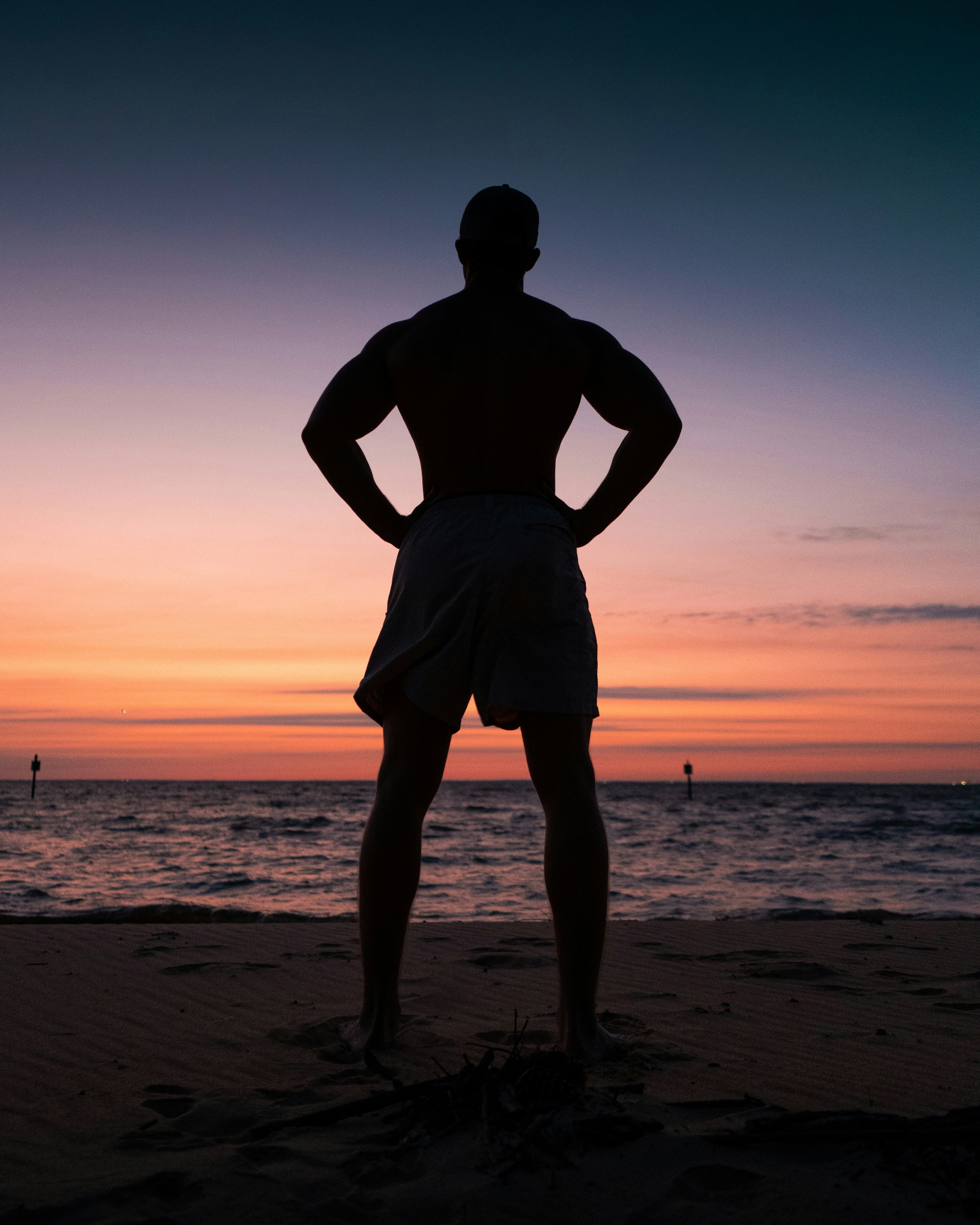
QuickFit app
Workouts that fit your schedule, goals, and lifestyle.
Workouts that fit your schedule, goals, and lifestyle.
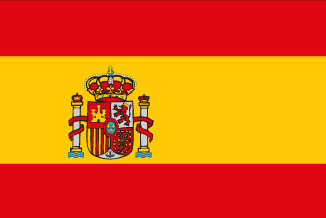
Quick translation
Homepage-subtitle
Workouts that fit your schedule, goals...
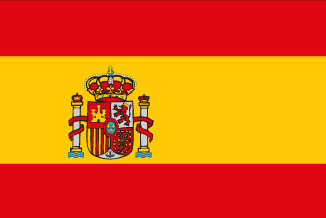
Entrenamientos que se adaptan a tu…
Tolgee native SDK
Tolgee native SDK provide the best developer and translator experience. Enhance in-context editing, context extraction or one-click screenshot generation.
TOLGEE CLI
Import & Export via UI
REST API & Webhooks
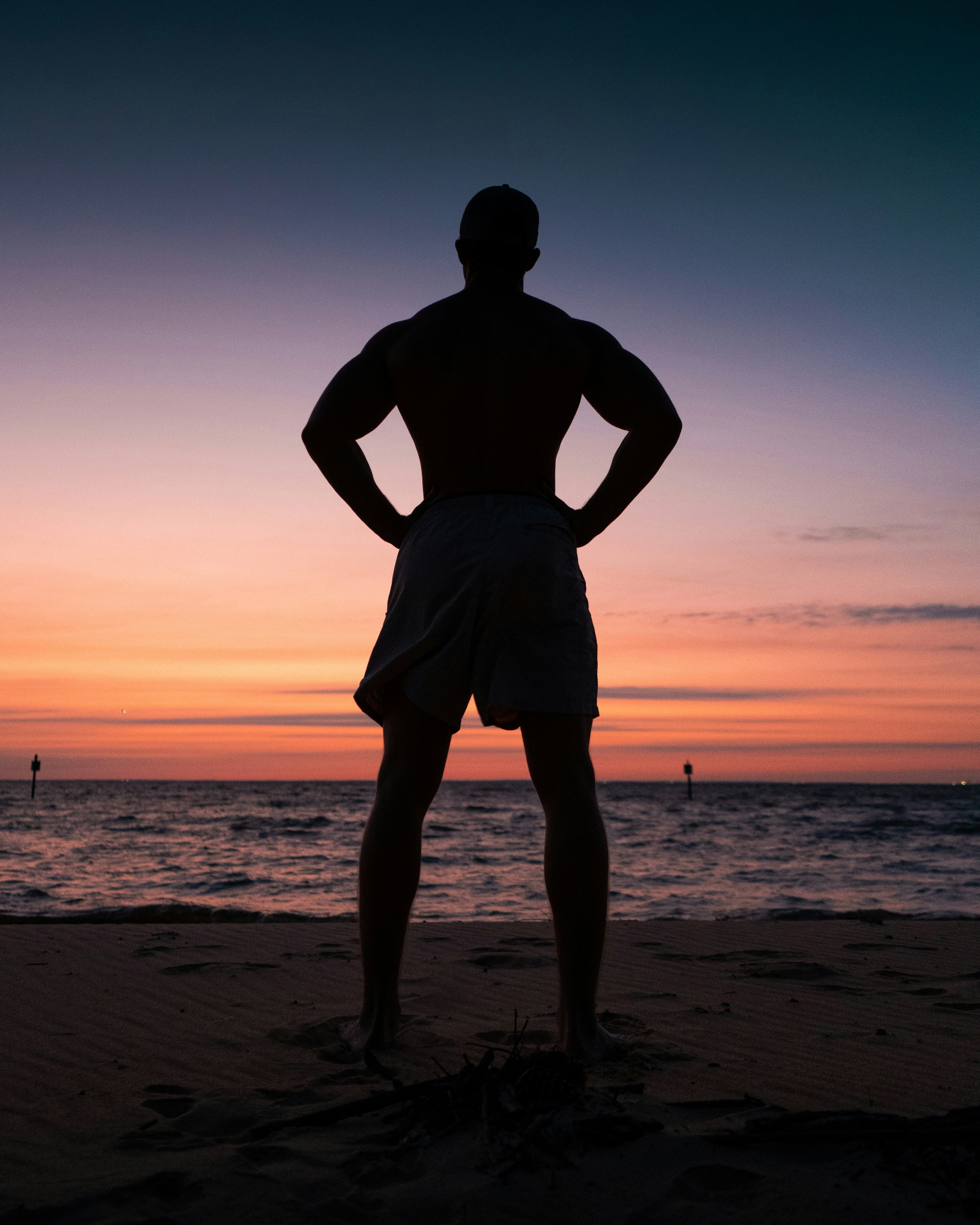
QuickFit app
Workouts that fit your schedule, goals, and lifestyle.
Workouts that fit your schedule, goals, and lifestyle.
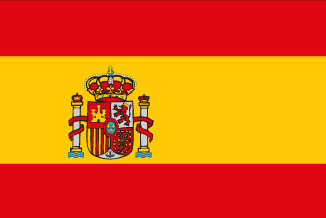
Quick translation
Homepage-subtitle
Workouts that fit your schedule, goals...
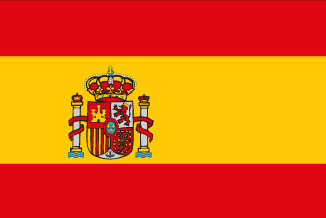
Entrenamientos que se adaptan a tu…
Tolgee native SDK
Tolgee native SDK provide the best developer and translator experience. Enhance in-context editing, context extraction or one-click screenshot generation.
TOLGEE CLI
Import & Export via UI
REST API & Webhooks
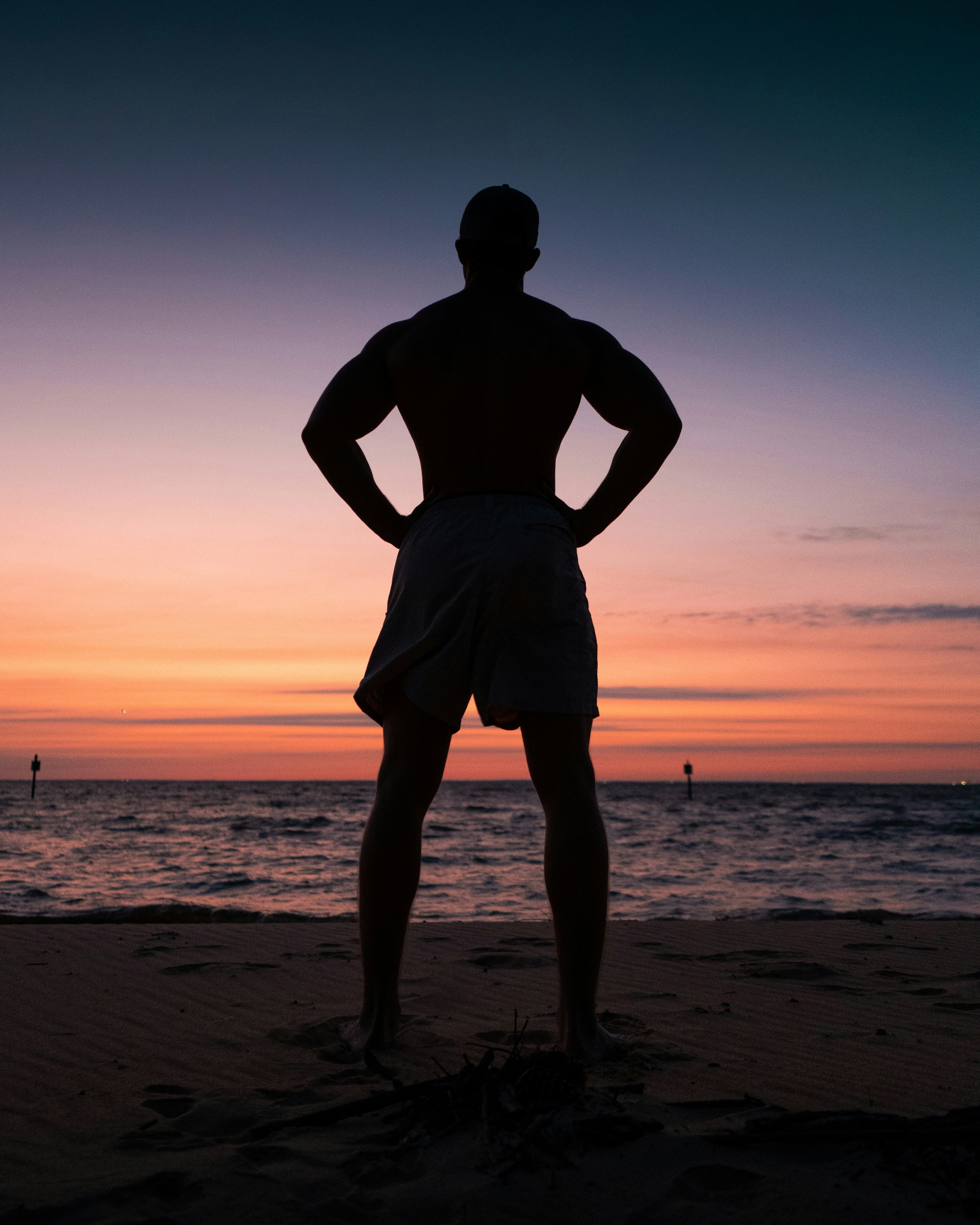
QuickFit app
Workouts that fit your schedule, goals, and lifestyle.
Workouts that fit your schedule, goals, and lifestyle.
Quick translation
Homepage-subtitle
Workouts that fit...
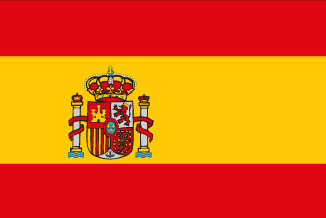
Entrenamientos que...
FAQ
How do I add new keys?
How do I add new keys?
How can I handle plurals?
How can I handle plurals?
Which machine translation providers are supported?
Which machine translation providers are supported?
How can product managers, designers or other non-developer team members edit the strings?
How can product managers, designers or other non-developer team members edit the strings?
Is my application dependent on Tolgee Servers?
Is my application dependent on Tolgee Servers?
How can I export the data for production?
How can I export the data for production?
Is my API key exposed in the production?
Is my API key exposed in the production?
What formats are supported by Tolgee?
What formats are supported by Tolgee?
What's the difference between development and production mode?
What's the difference between development and production mode?
Can I use strings created for web app for mobile and other apps?
Can I use strings created for web app for mobile and other apps?
How can I automate exporting data from the Tolgee Platform?
How can I automate exporting data from the Tolgee Platform?
Do you offer a completely free cloud plan?
Do you offer a completely free cloud plan?
Popular apps & integrations
We support all major JS frameworks. Set it up in minutes and keep working just the way you like - no disruptions, just smooth, hassle-free localization.
©
2025
Tolgee
All rights reserved.
©
2025
Tolgee
All rights reserved.